Python How to Use Function From Another File
Introduction
If you're new to Python and have stumbled upon this question, then I invite you to read on as I discuss how to call a function from another file. You have most likely used some of Python's built-in functions already like print()
and len()
. But what if you've defined your own function, saved it in a file, and would like to call it in another file?
Import it!
If you've ever imported something like random, NumPy, or math then it is really as simple as that! If you haven't, then here's a quick look at how it's done.
As an example, let's use the math module to find the square root of a number.
First, we import it.
>>> import math >>>
To see the available functions and attributes for a module use the built-in function dir()
:
>>> dir(math) ['__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc']
The function to calculate square root is called 'sqrt
'. And we'll use the dot notation to call it:
>>> math.sqrt(64) 8.0 >>>
Alternatively, you can use the keyword "from
" followed by the module name and "import
" followed by the attribute or function. This way we no longer have to use the dot notation when calling the square root function.
>>> from math import sqrt >>> sqrt(81) 9.0
And as expected, attempting to access the other functions or attributes still requires the dot notation:
>>> pi Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: name 'pi' is not defined >>> math.pi 3.141592653589793
User-Defined Functions
As you progress in your Python coding, you will eventually create your own functions and will implement them in other programs. As an example, we will illustrate this with a simple tip calculator. I invite you to follow along.
Open your favorite python editor. I'm currently using Linux so I'll just use vi for this example. I'll call my file "myfunctions.py
".

Here's the function definition:
def calcTip(b): # Tip will be 20% of the bill return (b * .2)
Save the file.
Now to call a function from another file in Python, we simply use "import" followed by the filename of your .py
file:
>>> import myfunctions >>> totalBill = 100.00 >>> tip = myfunctions.calcTip(totalBill) >>> print(tip) 20.0
If you have multiple functions in your file and would like to see them, don't forget to use the dir
function. In our case, it only shows the calcTip
function:
>>> dir(myfunctions) ['__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'calcTip'] >>>
Also, don't forget we can use the alternate method if you would like to skip the dot notation:
>>> from myfunctions import calcTip >>> totalBill = 250.00 >>> print(calcTip(totalBill)) 50.0 >>>
Things to watch out for
Note in my example, when I ran the Python interpreter it was within the same working directory as the myfunctions.py
file. If you're not familiar with Linux, the dollar sign is the command prompt.
- pwd = print working directory
- The current directory is "/home/pete/Videos/Python"
- ls -l = list directory contents
- The file "myfunctions.py" is located here
- python3 = invoke the python interpreter
- When entering the "import myfunctions" line, there is no error.
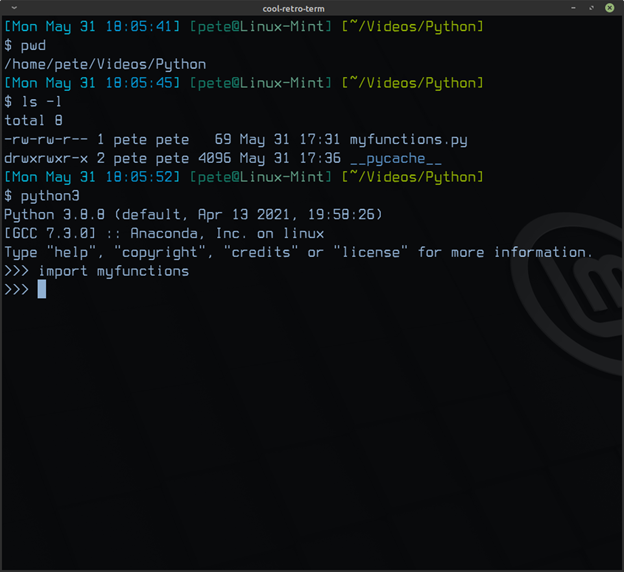
The below screenshot shows I change the working directory to home (~) and run pwd
to show the current directory path. I then run the python interpreter and attempt to import the myfunctions
file. Now it shows "ModuleNotFoundError
" because the file is not within the current directory.
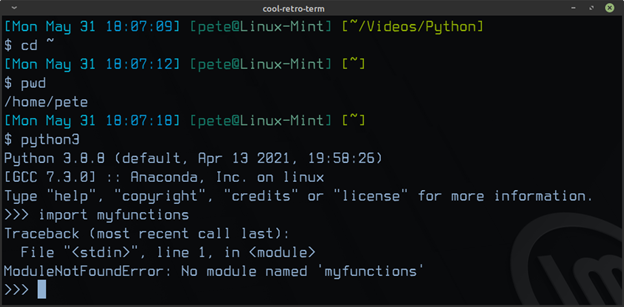
If you're using an IDE, then make sure your file is in your project. The below screenshot shows the Spyder IDE with a Project called "Function Example". Screenshot of "myfunctions.py
" file with the function definition of calcTip
:

Screenshot of the "main.py
" file. This particular IDE is really great because since the "myfunctions.py
" file is within our Project, then the autocomplete detects it when I import it.
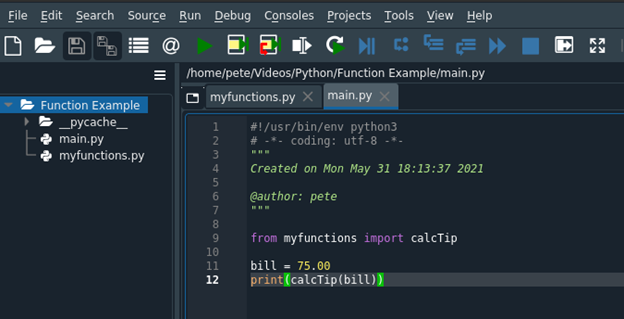
Here's the console output when running the main.py
file, passing variable "bill
" into the calcTip
function:

And that's how to call a function from another file in Python. I hope you found this article useful and look forward to writing more! See you soon!
Python How to Use Function From Another File
Source: https://blog.finxter.com/how-to-call-a-function-from-another-file-in-python/
0 Response to "Python How to Use Function From Another File"
Post a Comment